Hey @pim,
I am not quite sure how you mean that, but it is an open secret that AMD/Intel x86 APUs (CPU + GPU) are usually not so great in their GPU performance; one could also say they tend to suck big time on the GPU side. When we look at the AMD Ryzen 680M performance, we can see that the dirt cheap last generation entry level GeForce RTX 4060 Mobile is absolutely destroying it with 4.6 times its performance in the 3DMark 11. Or to make it tangible: A rendering which takes 10 minute on that last generation entry level GPU will take 46 minutes on that APU.
via notebookcheck.net:
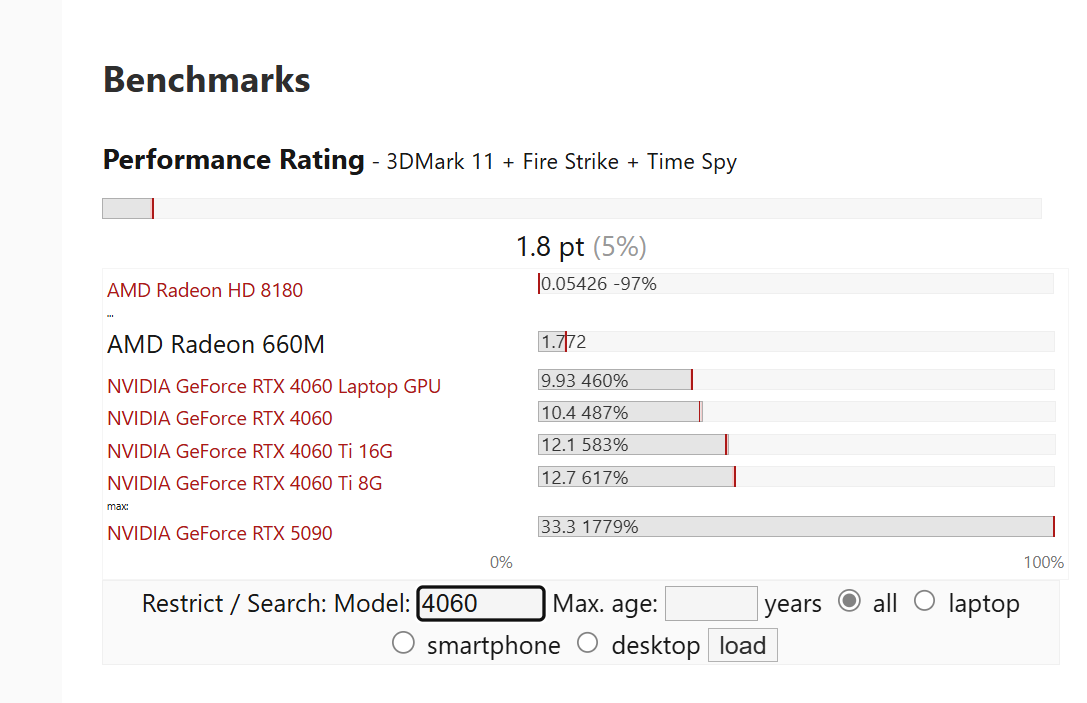
The 3D Mark performance is of course only indirectly important for Cinema 4D, as it directly only applies to the viewport, so my little tangible rendering comparison was a bit cheated. But it is indicative of the Cuda/OpenCL performance which is very important for rendering and simulation in Cinema 4D. notebookcheck is only using very old versions of Cinebench (only using the CPU), but I bet when you dig long enough, you will also find modern Cinebench 2024 scores (which use the GPU for rendering) for the AMD Ryzen 680M, and they will be horrible.
There are mini PC Windows builds which use dedicated mobile GPUs which will yield much better performance. They use mobile GPUs to fit into mini PC cases, and to keep their thermals in check, but the good builds tend to be very pricey. I would not buy anything under an RTX 4070 mobile GPU-wise for Cinema 4D. And even though Windows mini builds exist, they tend to be limited due to what is lined out below.
Because what cannot be overstated enough, is that the biggest issue with mini (x86) PC builds are the thermals. And your in theory super nice and shiny hardware will then be thermally throttled by 20%, 30%, or even 40% of its performance when some chip thinks after ten minutes of usage that it is getting too toasty. You can build very potent systems with x86/CISC architecture (Intel/AMD CPUs), which are also reasonably quite. But they are always huge (go under the desk and not on it), and you better not look at their power consumption and thermals. You can build mini x86 setups, but they then either sound like a starting rocket engine all the time (and such hot hardware also tends not to live very long) or get thermally throttled into oblivion. That is especially true for non-gaming rigs where you often want a lot of RAM and SSDs which worsen the heat problem.
The alternative is the ARM/RISC architecture because it is just better at being power efficient. Maxon currently only ships Cinebench for both the Apple and Windows ARM architecture. Cinema 4D is only shipped for the Apple ARM architecture, i.e., the M-series.
I have a quite beefy Windows workstation in the office. It is great in performance and reasonably quite, but has the size of a mini fridge and the power consumption of a small town. I very recently got my new Mac device for/from work, an M4 Mac mini 10C with 32GB of RAM, i.e., one of the lower end configurations. And this thing is an absolute unit when it comes to performance, fits into the palm of my hand, and has exceptional thermals and power consumption. I have not done any any concrete comparisons, and I think my Windows machine is still a bit more potent when push comes to shove, but the Mac mini gets VERY close for a fraction of the cost and size.
So, long story short: When you want a mini build, ARM is the way to go. But ARM has not yet fully matured on Windows and Maxon is currently not shipping Cinema 4D for ARM on Windows. Even as a long standing Apple non-appreciator, I have to admit that the M-series is an exceptional architecture with exceptional performance. When Apple is not an option, I would advise against a mini build, as there are currently simply no truly viable mini x86 builds which offer sufficient CPU and GPU performance for a 3D workstation. For an x86 3D workstation you are more or less forced to go with a big-box-under-the-desk build.
Cheers,
Ferdinand